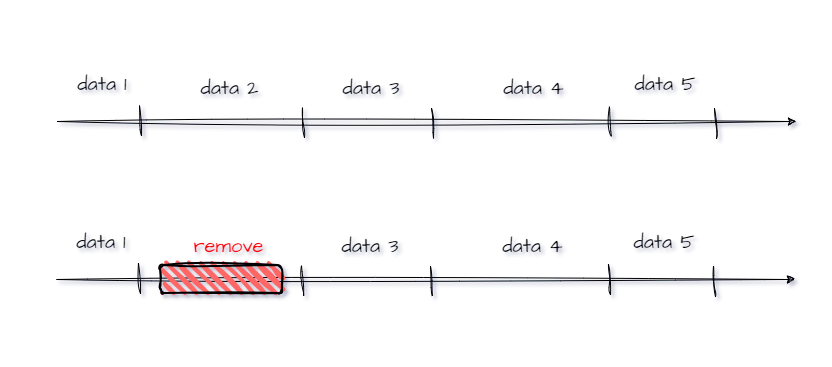
Một vài cách em hiện đang suy nghĩ:
- Dùng 1 byte để đánh dấu vùng nhớ đó đã bị xóa, thêm position vào 1 HashSet (Tracker). Khi thêm mới 1 Object sẽ kiểm tra Tracker và tìm vị trí có space đủ để ghi vào, tuy nhiên độ dài Object có thể ít hơn nên space của vùng nhớ đó nên vẫn bị trống 1 phần
- Dịch tất cả các Object phía sau lên tương tự như ArrayList, tuy nhiên em lại không biết cách set lại position cho HashTable