Share & grow the worlds knowledge!
We want to connect the people who have knowledge to the people who need it, to bring together people with different perspectives so they can understand each other better, and to empower everyone to share their knowledge.
stream
×
Remain: 5
1 Answer
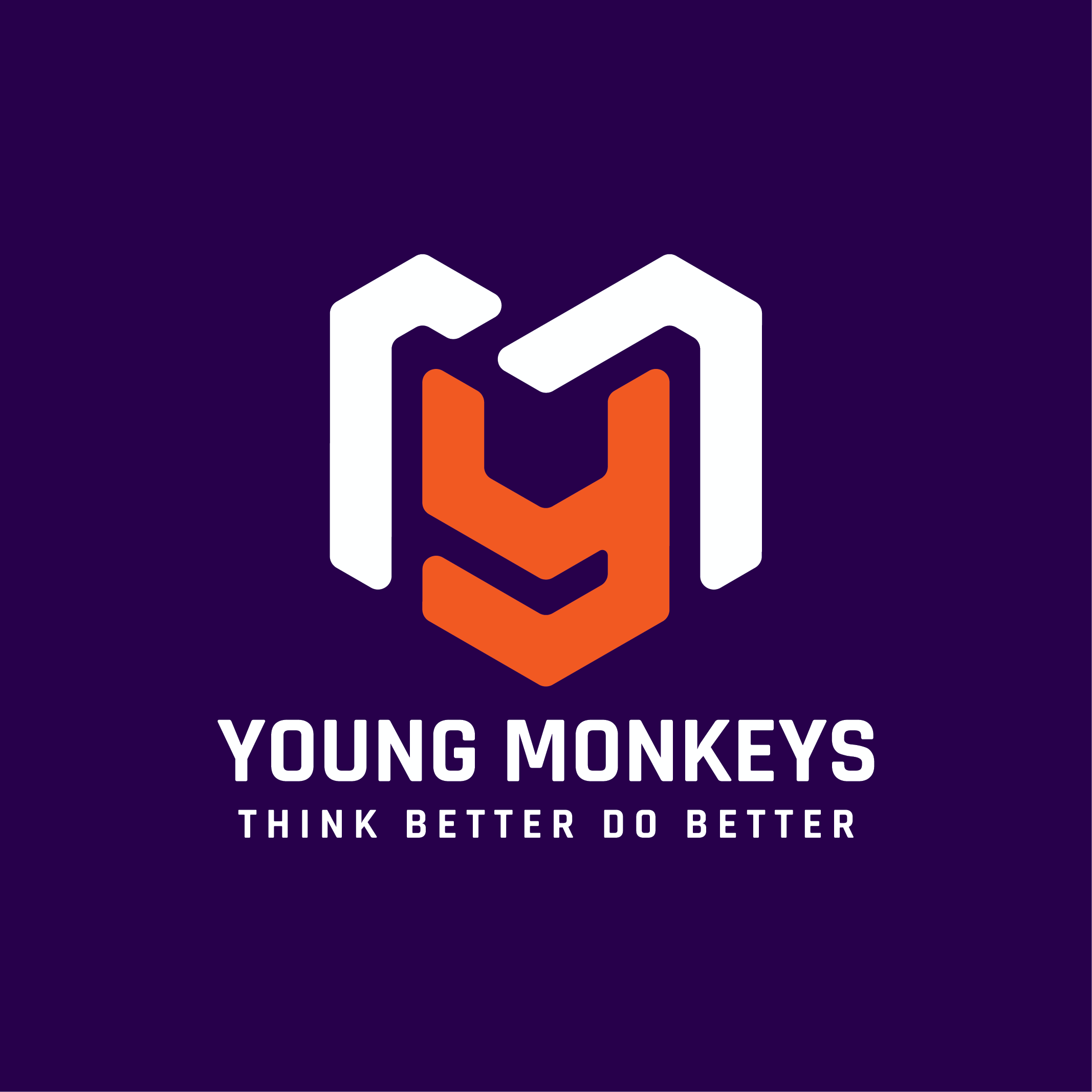
monkey
Enlightened
monkey
Enlightened
Bài toán thống kê lượt visit từ microservice và tổng hợp thành map userId - lượt visit, sử dụng java 8 stream:
import java.util.Arrays; import java.util.HashMap; import java.util.Map; import java.util.Objects; import java.util.Optional; import java.util.stream.Collectors; import lombok.AllArgsConstructor; public class VisitCounterExample { public static void main(String[] args) { Map[] visits = new Map[3]; visits[0] = new HashMap(); visits[0].put("1", new UserStats(Optional.of(10L))); visits[0].put("2", new UserStats(Optional.empty())); visits[0].put("3", new UserStats(Optional.of(10L))); visits[2] = new HashMap(); visits[2].put("1", new UserStats(Optional.of(10L))); visits[2].put("2", new UserStats(Optional.empty())); visits[2].put("3", new UserStats(Optional.of(20L))); System.out.println(new VisitCounterExample().count(visits)); } Map count(Map... visits) { return Arrays.stream(visits) .filter(Objects::nonNull) .map(userStatsById -> userStatsById .entrySet() .stream() .filter(it -> isNumber(it.getKey())) .filter(it -> it.getValue().getVisitCount().isPresent()) .collect( Collectors.toMap( it -> Long.parseLong(it.getKey()), it -> it.getValue().getVisitCount().get() ) ) ) .flatMap(it -> it.entrySet().stream()) .collect( Collectors.toMap( Map.Entry::getKey, Map.Entry::getValue, Long::sum ) ); } private static boolean isNumber(String str) { try { Long.parseLong(str); return true; } catch (NumberFormatException e) { return false; } } @AllArgsConstructor public static class UserStats { private Optional visitCount; public Optional getVisitCount() { return visitCount; } } }
-
0
- Reply
- Questions 1.0K
- Answers 2.2K
- Best Answers 135
- Users 510
-
-
-
u0ugfg1mx4sia624 added an answer cho mình hỏi cái token gửi lên cho viettel post, mình để đâu... 1746373212000
Related Questions
Recent Activities
-
Voted up question. January 9, 2023 at 2:54 pm
-
Voted up question. January 9, 2023 at 2:54 pm
-
Voted up question. January 9, 2023 at 2:54 pm
-
Voted up question. January 9, 2023 at 2:54 pm
-
Voted up question. January 9, 2023 at 2:54 pm
Top Members
Trending Tags
.net
.net core
.net oop
#formatdate
abstract class
access app
access token
ai
android
ansible
anti-flooding
apache poi
api
app
architecture
artificial intelligence
assembly
async
asyncawait
atomicboolean
authentication
backend
backend nestjs
background
bash script
batch
bean
big project
binding
bitcoin
blockchain
blog
boot-nodes
branch
btree
bucket4j
buffered
build
bundle
c#
c# .net
cache
caching
callback
career
career path
cast
câu hỏi
centos
chat
cloud
cloud reliability
commit
communication
computer science
concurrent
config-css
connection pool
content-disposition
contract
controllerservice
convert date to number
cookie
cors
cosmos
cosmos-sdk
crawl data
cron
css
database
database migration
datasource
datastructure
datetime
deadlock
debug
decentralized exchange
deep learning
deploy contract
design patterns
design-pattern
dev
devops
dex
di
distraction programing
dns
đồ án tốt nghiệp
docker
download
draw.io
du học
dữ liệu lớn
duration
eclip
editor
elasticsearch
email
erc20
erc721
estimation
eth
ethereum
ethereum login
excel
excel-object-mapper
exception
exception handle
exception handler
executor
export compliance
extensions
exyfox
ezyfox
ezyfox-boot
ezyfox-server
ezyfoxserver
ezyhttp
ezyjpa
ezymq-kafka
ezyplatform
ezyredis
facebook
fe
filter
floating point
flutter
format json
freetank
front-end
frontend
fullstack
fulltextsearch
future
gallery
game
game-box
game-room
game-server
gateway
get
get file zip
git
glide
go
golang
gorilla
graduation thesis
graphql
grapql
grpc
guide
h2 database
handy terminal
hazelcast
hibernate
hibernateconfig
html
http
https
hyperloglog
image
index
indexing
integration-test
intellij
interface
io
ioc
ipfs
isolate
issue
it
java
java core
java spring
java web
javacore
javascript
javaw
jenkins
jetbrains
job
join
jotform
jpa
jquery
js
json
json file
json to object
jsonproperty
jsp
jsp & servlet
junit-test
jvm
jwt
kafka
keep promise
kerberos
keycloak
kotlin
languague
laravel
library
list
load balancer
load-balancing
lock
log
log4j
log4j-core
login
lỗi font
lưu trữ
machine learning
macos
mail
mail template
main
map
maria db
math
maven
merge
message queue
messaging
metamask
microservice
microservices
migration
mobile
model
mongo
monitoring
mq
msgpack
multi-threading
multiple tenant
multithread
multithreading
mysql
n
naming
naming convention
nan
netcore
netty
network
networking
nft
nft game
nginx
nio
node.js
nodejs
non-blocking io
null
oop
opensource
optimize
oracle
orm
otp message
paginaiton
pagination
pancakeswap
panic
partition
pdf
pgpool
phỏng vấn
php
plugin
pointer
postgresql
postman
pre
private_key
procedure
profile
programming
programming-language
project management
promise
properties
push message android
push notification
push-noti
python
python unicode
qr
qrcode
question
queue
rabbitmq
react-native
reactive
reactjs
reactjs download
readmoretextview
recyclerview
redis
refactor
refresh token
regex
replica
repository
request
resilence4j
resource
rest
restful
resttemplate
roadmap
room database
ropssten
ropsten
round robin
rust
rxjava
s3
schedule
scheduled
scheduled spring boot
search
security
send email
send mail
serialization
server
servlet
session
shift jis
singleton
sjis
slack
smart contract
soap
socket
socket server
soft delete
solution
sosanh
spring
spring aop
spring boot
spring data jpa
spring redis
spring security
spring websocket
spring websocket cors
spring-boot-test
spring-jpa
springboot
springsecurity
springwebflux mysql
sql
sql server
sse
ssl
ssl email
stackask
storage
stream
stream api
stress test
stresstest
structure trong spring boot
study
synchronize
synchronized
system environment variables
tcp
test
thiết kế tầng trong dự án
thread
thread pool
threadjava
threadpool
thymeleaf
tomcat
totp
tracking location
transaction
transfer
transfer git
udp
uniswap
unit test
unity
upload
upload file
utf-8 file
validate
validate date
vector
video call
vietqr
view
volatile
vue
vue cli
vuejs
watermark
web
web3
web3 client
webassembly
webflux
webpack
webrtc
websocket
windows 11
winforms
wordpress
work
xss
zip file
zookeeper